We all want things to "just work." It’s easy to take for granted how seamlessly our smart devices connect—whether it’s asking Alexa to start the vacuum or setting an automated schedule for the lawnmower. But when it comes to business processes, things aren’t always so smooth. Many systems still require manual effort to transfer data between platforms, leaving gaps that slow things down. One way to close these gaps is through integration, and APIs provide the key to making systems communicate effortlessly. Once you have some little tricks up your sleeves, things become so much easier.
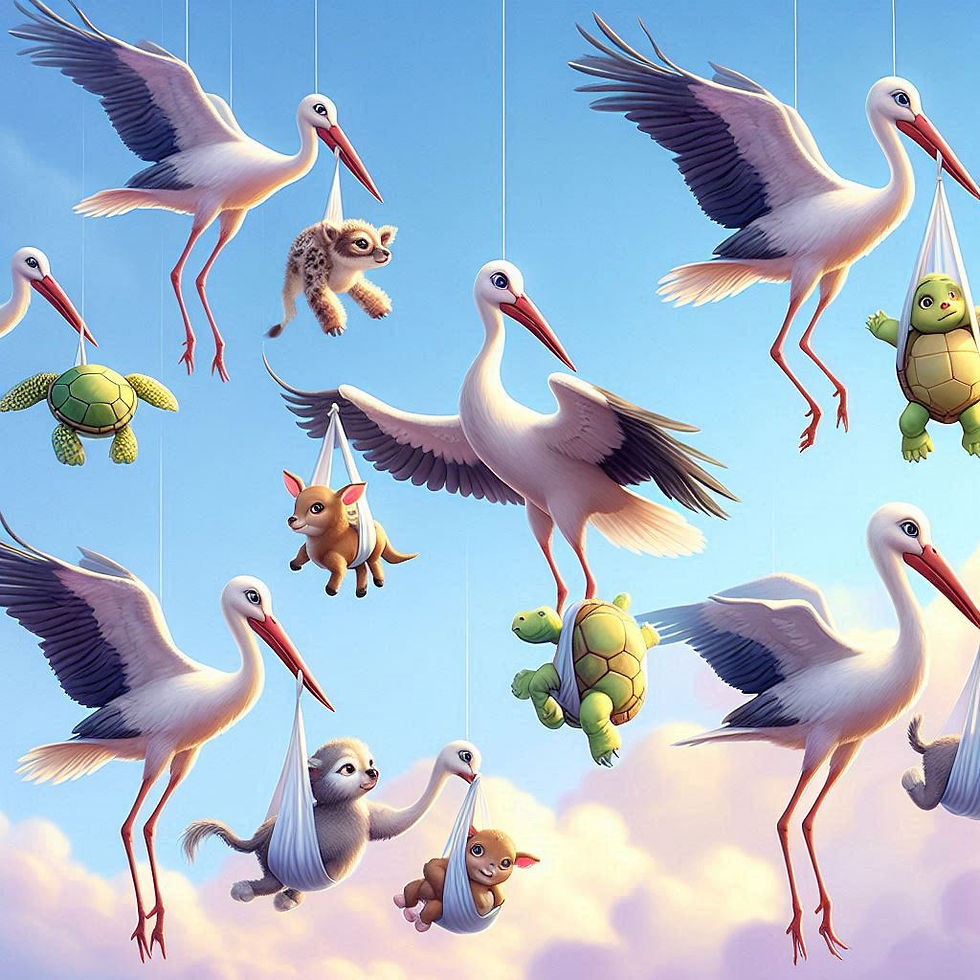
Why you should Use Dynamic Parameters in API Calls
Hardcoding API queries may work in small-scale scenarios, but when handling frequently updated datasets, this approach quickly becomes impractical. Dynamic parameterisation allows:
Automated data retrieval without manual intervention.
Up-to-date information from external sources.
Custom API queries based on user input or business logic.
Scenario: Inventory Management System API Query in Power Query
Consider an inventory management system that provides product stock data via API. The objective is to retrieve stock availability for multiple products based on category and date range dynamically.
Sample API Endpoint
This endpoint requires:
ProductID – The unique identifier for the product.
Query parameters:
category – The category to filter the products.
date_from – The start date for checking stock levels.
date_to – The end date for checking stock levels.
_cacheBuster – A unique value to ensure fresh data.
Constructing the API Query Dynamically in Power Query
Using Power Query, we can iterate over a dataset of products and dynamically build API requests.
Example in Power Query
let
BaseUrl = "https://api.inventoryhub.com/v1/products/",
Products = {"A123", "B456", "C789"}, // Example Product IDs
Category = "electronics",
DateFrom = "2024-01-01",
DateTo = "2024-02-01",
GetStockData = (ProductID as text) =>
let
QueryParams = [
category = Category,
date_from = DateFrom,
date_to = DateTo,
_cacheBuster = Number.ToText(Number.RoundDown(Number.From(DateTime.LocalNow()) * 1000))
],
ApiUrl = BaseUrl & ProductID & "/stock",
RawResponse = try Json.Document(Web.Contents(ApiUrl, [Query = QueryParams])) otherwise null
in
RawResponse,
StockDataList = List.Transform(Products, each GetStockData(_)),
StockDataTable = Table.FromList(StockDataList, Splitter.SplitByNothing(), {"StockData"})
in
StockDataTable
Breaking Down the Approach
Loop Through Product IDs – Instead of making individual static calls, multiple products are processed using List.Transform.
Dynamic Query Parameters – Real-time variables are injected into the API request.
Cache Busting – The _cacheBuster parameter ensures that the request fetches fresh data by appending a timestamp.
Error Handling – Using try ... otherwise null prevents the query from breaking due to failed API calls.
Note: We use a static product list here but you can easily put this into your existing query and pass the products in form your table.
Real-World Use Cases
E-commerce: Automating stock level updates across multiple warehouses.
Logistics: Querying shipment statuses dynamically based on package IDs.
Finance: Retrieving up-to-date stock prices for multiple assets in one go.
Final Thoughts
Dynamic API queries in Power Query improve automation and scalability. Whether working with inventory, finance, or logistics data, implementing an adaptable approach reduces manual effort and increases efficiency.
Next time you work with an API in Power Query, consider how dynamic parameterisation could simplify your workflow. Happy querying!